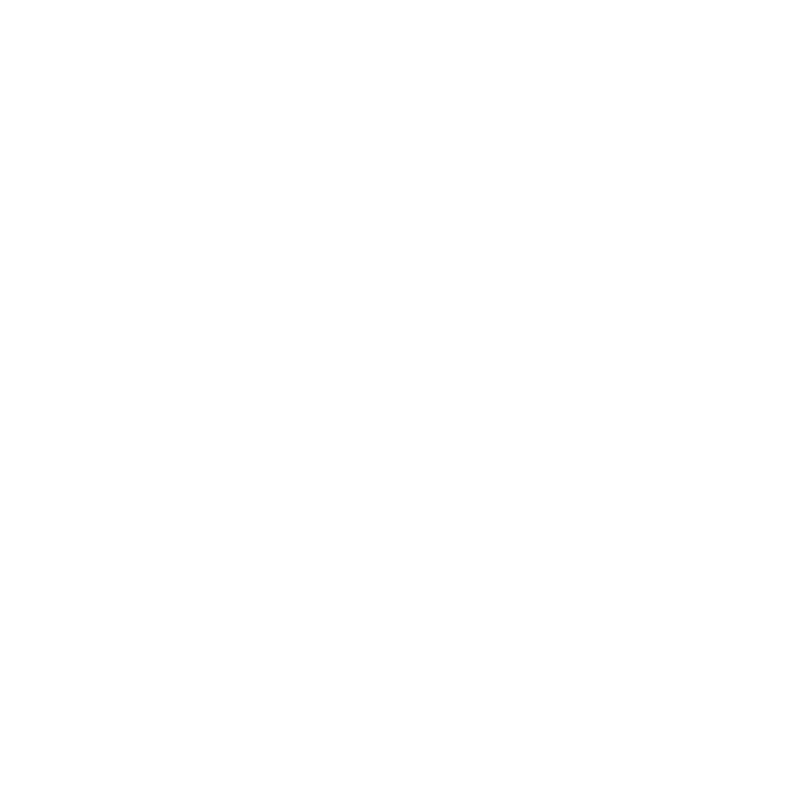
TABLE OF CONTENTS
Cross-platform 3D Rendering in Flutter
Flutter does not have native 3D rendering support out-of-the-box, but it can be extended to render 3D graphics using external libraries and plugins. To build a Three.js-like 3D library for Flutter, we need to consider existing solutions, rendering technologies (WebGL, Vulkan, OpenGL), language interoperability, performance, and cross-platform challenges. Below is a structured overview of how such a library can be implemented, including current tools, best practices, and recommendations.
Flutter does not have native 3D rendering support out-of-the-box, but it can be extended to render 3D graphics using external libraries and plugins. To build a Three.js-like 3D library for Flutter, we need to consider existing solutions, rendering technologies (WebGL, Vulkan, OpenGL), language interoperability, performance, and cross-platform challenges. Below is a structured overview of how such a library can be implemented, including current tools, best practices, and recommendations.
Existing 3D Libraries for Flutter and Limitations
Several community-driven projects have attempted to bring 3D rendering to Flutter. Key examples include:
- three_dart – A Dart port of the popular Three.js engine. It implements Three.js API in pure Dart and relies on a plugin called
flutter_gl
to access OpenGL/WebGL for rendering. It supports Android, iOS, web, Windows, and macOS (Linux support is still a TODO). Limitations: As a port of Three.js (currently based on r138), it may lag behind the latest Three.js features and performance improvements. Also, heavy computation in Dart and frequent FFI calls for every WebGL operation could impact performance compared to a native engine.
- Flutter Scene (flutter_scene) – A new real-time 3D engine written for Flutter, originally derived from Flutter’s Impeller codebase. It’s a pure Dart library that uses Flutter’s low-level GPU rendering API (in preview) and works only when Flutter’s Impeller engine is enabled. It aims for high performance and easy integration with Flutter UI. Limitations: It is in early preview and not yet stable. Being tied to Flutter’s evolving GPU API means it may require using the master channel of Flutter and might break as APIs change.
- Flame Engine (flame_3d) – Flame is a Flutter game engine primarily for 2D, but experimental 3D support is being explored via
flame_3d
. The Flame team has indicated they plan to add true 3D once Flutter’s Impeller and shader support are fully stable. Limitations: As of early 2024, this is still experimental. Flame’s maintainers noted the need for depth buffer (Z-buffer) access and better shader APIs in Flutter to build a “good 3D game engine”, which were not fully available at the time.
- Unity and Unreal integration – Rather than a Flutter-specific 3D library, some projects embed existing game engines. For example, the Flutter Unity Widget allows embedding a Unity 3D scene in a Flutter app. Limitations: This approach runs an entirely separate engine (Unity) alongside Flutter. It offers powerful 3D capabilities but comes with significant overhead – large app size, higher memory/CPU use, and complexity in the integration. UI overlays and interactions between Flutter and the 3D view can be limited, since Unity renders to a native view or texture.
- Filament (Thermion) – Filament is an open-source, cross-platform PBR (physically-based rendering) engine by Google. The Thermion project provides Flutter/Dart bindings to Filament. It uses Flutter plugins and FFI to render with Filament on mobile and desktop, and even includes a WebAssembly/WebGL fallback for web browsers. Limitations: Integrating a complex C++ engine requires maintaining platform-specific binaries and dealing with large native libraries. Thermion is quite advanced, but as of its announcements, web support was “hacked-together” and Linux support was pending, indicating ongoing development. Using Filament also means adopting its architecture and possibly being constrained by its update cycle.
Each of these solutions demonstrates that cross-platform 3D in Flutter is feasible, but often with trade-offs. No single library has yet emerged as a de-facto standard, largely because of the challenges in balancing performance, API stability, and platform coverage.
WebGL for Web-Based Rendering
To support rendering in Flutter web, WebGL is the primary path (since browsers do not allow direct Vulkan or native OpenGL). There are a few approaches to integrate WebGL in a Flutter web app:
- Use a WebGL Canvas via a Flutter plugin: Flutter web can interoperate with HTML elements when using the HTML renderer. A plugin can create an HTML
<canvas>
element and use JavaScript or Dart (viadart:html
) to call WebGL APIs. For example, theflutter_gl
plugin does exactly this – on web, it inserts a WebGL canvas and provides a Dart interface that mirrors the WebGL API. The library’s API is intentionally made similar to WebGL, so the same Dart code can work on web (calling actual WebGL in the browser) and on mobile/desktop (calling OpenGL via FFI). This unified API approach allows writing code once for all platforms.
- Dart JS Interop or CanvasKit: If using Flutter’s CanvasKit (which is WebAssembly-based Skia) for other UI, one might still create a separate WebGL context for the 3D content. Direct JS interop calls from Dart can invoke WebGL commands in the browser. However, managing a separate rendering loop in JavaScript alongside Flutter’s rendering may require careful coordination (e.g., to ensure the canvas is correctly overlaid and updated).
- WebAssembly for heavy logic: For performance, intensive 3D computations or existing engines can be compiled to WebAssembly for the web. In the Filament integration example, the engine core is compiled to WASM and then used with WebGL2 in the browser. The Flutter app can communicate with that WASM module either via JavaScript interop or package it as part of the app. This approach allows reusing native code on web, but adds complexity in data passing and setup.
Using WebGL on the web means targeting WebGL 2.0 (which is roughly equivalent to OpenGL ES 3.0) for better performance and features (like VAOs, instanced rendering, etc.). The library should gracefully handle absence of WebGL2 (fallback to WebGL1 if needed, or inform the user/browser requirements). To maximize compatibility, sticking to WebGL’s constraints (e.g., shader language GLSL ES, and no compute shaders as in WebGPU) is necessary. As WebAssembly and WebGPU mature, future versions of the library could consider WebGPU for better performance, but as of now WebGL ensures broad compatibility.
Vulkan as Primary Rendering API (with OpenGL Fallback)
On mobile and desktop platforms, Vulkan offers modern, high-performance graphics, and should be used where available. Many new or high-end devices support Vulkan, but a fallback to OpenGL is essential for broader coverage:
- Advantages of Vulkan: Vulkan is a low-overhead API that provides more direct control over the GPU, better multi-threading, and reduced driver overhead compared to OpenGL. Properly used, it can yield more consistent frame rates and take advantage of multiple CPU cores for preparing rendering work. It’s designed for modern GPUs and is the primary path for forward-looking development (for example, Flutter’s Impeller engine leverages Vulkan on Android by default).
- Need for OpenGL fallback: Not all devices or platforms support Vulkan. For instance, older or low-end Android devices (especially those pre-2016) might lack Vulkan support or have incomplete drivers. Similarly, Flutter on Linux might run in environments without Vulkan drivers. In these cases, falling back to OpenGL ES (on mobile) or OpenGL (desktop) ensures the app can still run. This is exactly how Flutter’s engine behaves: on Android, Impeller uses Vulkan if available and automatically falls back to the older OpenGL renderer on devices that don't support Vulkan. A similar strategy can be applied in a custom 3D library.
- Platform-specific APIs: On iOS and macOS, Vulkan isn’t natively available. Apple platforms favor Metal (Apple’s graphics API). To use Vulkan there, a tool like MoltenVK can translate Vulkan calls to Metal. MoltenVK effectively lets Vulkan “run” on iOS/macOS by implementing Vulkan 1.2 on top of Metal. This means a Vulkan-based engine can be made to work on Apple devices via an extra layer. However, using MoltenVK adds a dependency and some overhead; an alternative is to implement a Metal backend specifically for those platforms (as engines like Filament or Unreal do). Another fallback on iOS could be OpenGL ES 3.0 (which, while deprecated by Apple, is still available through iOS 14 and 15 in practice). In summary, for all Flutter platforms: Vulkan can cover Android, Windows, Linux, and potentially macOS/iOS via MoltenVK, while OpenGL covers legacy devices and web (WebGL), and Metal could be considered for best performance on Apple hardware.
- Implementing multi-backend support: Designing the library to support two graphics APIs can be done in two ways. One approach is to use a graphics abstraction layer – for example, writing the engine with an abstraction that can be implemented by a Vulkan renderer and an OpenGL renderer interchangeably. This increases development effort (essentially writing and maintaining two rendering paths), but libraries like bgfx or wgpu can simplify this. bgfx is a C++ library that abstracts graphics backends (it supports DirectX, OpenGL, Vulkan, Metal, etc.), allowing you to write rendering code agnostic to the API. wgpu (a Rust/WebGPU-based framework) similarly can target Vulkan, Metal, DirectX, or WebGL, from a single codebase. Using such an abstraction could dramatically ease the support for multiple backends at the cost of adding an external dependency. The other approach is to primarily implement Vulkan and include a minimal OpenGL ES path for compatibility – basically only using OpenGL when Vulkan isn’t available. This is simpler initially, but you must ensure all major features (shaders, pipeline setup, etc.) have equivalents in OpenGL. Given Vulkan and OpenGL have different paradigms (e.g., Vulkan has explicit pipeline objects and descriptor sets, while OpenGL uses implicit state and global binds), an abstraction layer or separate modules might be necessary to keep the code manageable.
Overall, prioritizing Vulkan means the library will be “future-proof” and efficient on modern hardware, but robust fallback logic is needed. This includes detecting Vulkan support at runtime (or compile-time for a given platform) and gracefully switching to OpenGL. It’s worth noting that Vulkan’s advantages come with complexity: writing Vulkan rendering code is more verbose and requires managing more details (like memory, synchronization) than OpenGL
. This suggests that critical Vulkan code may be better handled in a native language (C++/Rust) for performance and safety, rather than in Dart.
Low-Level Languages and Flutter Interoperability (C++ vs Rust)
To achieve high performance, especially for the Vulkan and OpenGL rendering implementations, using a low-level language like C++ or Rust is advisable. Flutter provides two primary mechanisms to use such languages: platform channels and dart:ffi (Foreign Function Interface). For a 3D rendering library aiming to be cross-platform, dart:ffi is the more direct and efficient route, as it allows calling native code from Dart without going through platform-specific message channels.
- C++ integration: C++ is widely used in graphics (most engines and APIs provide C/C++ interfaces). A C++ rendering engine can be compiled into a dynamic library (.so for Android/Linux, .dll for Windows, .dylib or framework for iOS/macOS). Using Dart FFI, Flutter apps can load this library and call its functions directly. This approach has been successfully used in projects like Flutter’s own engine (which is C++), and community plugins wrapping C++ libs (e.g., SQLite, OpenCV, or Filament via Thermion). Interoperability is achieved by exposing C ABI functions (
extern "C"
) in the library for all the functions Dart needs to call. On the Flutter side, one would write Dart bindings (possibly usingpackage:ffi
andffi:calloc
for memory) to call these functions. The new Dart “native assets” feature can streamline bundling such native libraries with a Flutter package (allowing a pub package to include precompiled binaries or build scripts). C++ has the benefit of being natively performant and has mature tooling for graphics (e.g., you can utilize existing Vulkan or OpenGL headers, plus libraries like glm for math). The downside is dealing with memory safety (bugs in C++ can crash the app) and the need to compile separately for each platform (including armv7, arm64, x64, etc.).
- Rust integration: Rust offers memory safety and a modern ecosystem, which can be very beneficial for a complex engine. Rust can interoperate with C via FFI easily – you can write a Rust library and expose C-callable functions (using
extern "C"
and perhaps thecbindgen
tool to generate headers). From Flutter’s perspective, calling Rust code is the same as C/C++ code via FFI. Rust has strong community support for graphics: projects like gfx-rs/WGPU (which Rust uses for WebGPU and can target Vulkan, Metal, OpenGL), Ash (a Vulkan bindings crate), and others could jump-start development. For example, one could write the rendering core in Rust using wgpu for multi-backend support, then compile it to native and WASM targets. This strategy could allow a single Rust codebase to cover Android, iOS, desktop (native code using Vulkan/Metal/GL via wgpu), and web (WASM using WebGL/WebGPU). Interoperability gotchas include data passing – large data transfer between Dart and Rust should be minimized (perhaps by sharing pointers or using memory mapped assets) and threading – Rust code can spawn its own threads for rendering, but one must be careful not to violate Flutter’s threading rules for UI updates. There are also higher-level tools like flutter_rust_bridge, which generate bindings to call Rust from Dart more ergonomically, but those might add overhead. A manual FFI integration can be more efficient for performance-critical frames (e.g., calling a singlerenderFrame()
function each tick, implemented in Rust/C++, which internally handles all the draw calls).
- Managing Web compatibility: Neither C++ nor Rust code can run directly on the web, so a web strategy is needed. If the core engine is in C++, Emscripten can compile it to WebAssembly and JavaScript. If in Rust, it can compile to
wasm32-unknown-unknown
target (possibly usingwasm-bindgen
orstdweb
for WebGL calls). The Flutter web app could then load that WASM module. Projects like Thermion have already demonstrated a working (if experimental) path for a C++ engine via WASM. Another option is to maintain a parallel Dart implementation for web (as three_dart does), but that doubles the work. A practical recommendation is to abstract the rendering logic behind an interface and provide two implementations: one in native code (C++/Rust) for mobile/desktop, and one in Dart (or WASM) for web. The Dart implementation can be simpler or have reduced features if needed, just enough to visualize something in the browser. This way, when running on web, you avoid FFI (which is not available on web) and instead rely on Dart’s direct access to WebGL or on calling into WASM via JavaScript.
- FFI overhead and considerations: Dart FFI is quite fast for calling native functions, but there is some overhead per call (roughly on the order of a few microseconds). This means that calling thousands of small functions per frame (e.g., each individual OpenGL call via FFI) can become a bottleneck. It’s better to batch work in the native side. For instance, instead of calling a Dart FFI function for every vertex, one could pass large vertex buffers to native memory once and then invoke a single draw call. The
flutter_gl
project notes that converting a DartList
to an FFI pointer requires a memory copy, so they introduced NativeArray types to avoid repeated copying. Techniques like that or using persistent mapped buffers can help. Also, the FFI calls can be asynchronous if using Dart isolates or by having the native side run its own thread and sync with Dart via events, which can keep the Dart UI thread free. Both C++ and Rust can manage their own memory and threads; the Flutter library would need to ensure proper synchronization (for example, only update the Flutter UI from its UI thread, but heavy 3D logic can run on a separate thread).
Summary: Choosing C++ vs Rust largely comes down to developer preference and available libraries. C++ might integrate a bit more straightforwardly with existing graphics code (and can directly use Vulkan/GL headers or even reuse engines like OGRE, BGFX), whereas Rust offers safety and a unified path to WASM. Both can achieve the goal. In either case, Dart’s role would be to provide the high-level Flutter integration (like widget embedding, event handling, maybe scene management classes), while the low-level rendering and math heavy-lifting occur in the native layer for performance
. This hybrid approach leverages Flutter for UI and platform portability, and native code for optimized 3D rendering.
Performance Optimization Techniques for Real-Time 3D
Rendering 3D scenes at interactive frame rates (60 FPS or more) on multiple platforms requires careful optimization. Key techniques include:
- Efficient Rendering Pipeline: Minimize state changes and draw calls. Batching draw calls when possible (drawing many objects in one call via instancing or merging meshes) reduces overhead. Use Vertex Buffer Objects (VBOs) and keep data on the GPU to avoid re-uploading each frame. Both OpenGL and Vulkan reward submitting larger chunks of work rather than many small ones.
- Level of Detail (LOD) and Culling: To maintain performance, especially on mobile, implement frustum culling (don’t render objects not currently visible in the camera’s view) and possibly occlusion culling (skip objects hidden behind others). Use simpler models (lower polygon count or imposter sprites) for objects far from the camera. This reduces the vertex processing load. Techniques like quad-trees/octrees or BSP trees can organize objects spatially for quick culling lookups.
- Optimized Shaders: Write GLSL/HLSL shaders efficiently – avoid heavy calculations in fragment shaders (which run per pixel) if a vertex shader or precomputed texture could do it. Utilize lighting approximations like normal mapping instead of high-poly geometry. Precompile shaders when possible. Flutter’s Impeller, for example, precompiles a known set of shaders to avoid runtime compilation jank. In a custom engine, you can compile or load SPIR-V (for Vulkan) or GLSL (for GL) at initialization and reuse them. Also leverage shader features like UBOs (uniform buffer objects) to update many shader parameters in one block rather than setting individual uniforms repeatedly.
- Parallelism and threading: Take advantage of multi-core CPUs by preparing work in parallel. Vulkan is designed for multi-threading – command buffers can be recorded on worker threads while the main thread submits them. If using OpenGL, you are more constrained (OpenGL ES is typically single-threaded in practice), but background threads can still be used for asset loading, animation updates, AI, etc., leaving the main thread to only issue draw commands. The engine could have a separate thread for the rendering loop, especially if using FFI; that thread runs the native rendering while the Dart UI thread remains responsive. Impeller’s design shows the benefit of concurrency, being able to distribute workloads across threads.
- Memory Management and Pooling: Reuse objects and memory to avoid GC pauses or allocation overhead. For example, reuse command buffers or framebuffers instead of creating new ones each frame. Use object pools for frequently created temporary objects (like math vectors) to reduce Dart garbage collection. On the native side, allocate large buffers once (for vertices, indices, uniforms) and update them in place each frame instead of reallocating. Also, free GPU resources (textures, buffers) promptly when no longer needed to avoid memory leaks that could slow down or crash the app over time.
- Profiling and Tuning: Use profiling tools on each platform to find bottlenecks. For instance, on Android, use systrace or GPU Inspector to see if you are bound by CPU (too many draw calls) or GPU (shader too slow, fill-rate issues). On web, use browser dev tools WebGL profiler or Spector.js. Optimize based on these insights – e.g., if fill-rate (pixel shader cost) is an issue, reduce overdraw (don’t draw layers of nearly-opaque objects on top of each other unnecessarily, perhaps by ordering draws or using depth pre-pass techniques). If CPU is the bottleneck, see if you can move more logic into shaders or batch more.
- Use of Efficient Data Structures: In managing the scene and game logic, choose structures that are cache-friendly. For example, store vertex data in interleaved arrays to improve memory locality. Use contiguous storage (like Dart’s
Uint16List/Float32List
or native arrays) for large data to benefit from vectorization. Leverage SIMD if available (e.g., Dart’sFloat32x4
or by using SIMD intrinsics in C++/Rust) for math-heavy calculations on positions or physics.
- Graphics API specific optimizations: Tailor some optimizations to each API. For Vulkan, use Descriptor Sets efficiently (bind once, draw many objects using a descriptor set with arrays of textures if possible) and avoid re-creating pipeline objects every frame. For OpenGL, minimize expensive calls like state queries or shader recompilations during rendering. Use double-buffering or triple-buffering for smoothness (Flutter’s engine by default uses double-buffering with vsync). Also consider render passes or techniques like deferred rendering if the lighting complexity in the scene grows (deferred rendering trades memory for the ability to handle many lights more efficiently by doing lighting in a second pass).
By applying these techniques, the library can achieve near-native performance. Notably, performance tuning is an ongoing process: different devices may have different bottlenecks (some GPUs handle lots of small draws well, others prefer few big draws), so allowing configuration and making the engine adaptive can be helpful (for instance, adjust LOD distances based on detected device capability). The goal is to maintain a smooth frame rate and responsive experience even as the scene complexity scales up.
Potential Challenges and Solutions
Developing a cross-platform 3D library in Flutter comes with a set of challenges. Below are some major challenges and how to address them:
- Cross-Platform API Differences: Each graphics API (WebGL, Vulkan, OpenGL, Metal) has its own nuances and available features. Vulkan is explicit with manual memory management, whereas OpenGL has driver-managed resources and a different shading language (GLSL vs. Vulkan’s SPIR-V or HLSL). Solution: Introduce an abstraction layer in the engine design to separate “what to render” from “how to render on this API.” This could mean writing a thin wrapper for common tasks (creating a texture, drawing a mesh) that has separate implementations for Vulkan and OpenGL. Using existing cross-platform render libraries (like bgfx or wgpu) is a practical shortcut to handle this at the cost of an extra dependency. For Metal (iOS), either use a Vulkan-to-Metal translator (MoltenVK) or write a Metal backend if you have the expertise. Testing on all platforms frequently is important to ensure consistency.
- Flutter Integration and UI Overlays: Unlike a standalone game engine, a Flutter 3D library must coexist with Flutter’s UI widgets. This can be tricky when trying to overlay Flutter widgets on top of 3D content (e.g., placing buttons or labels within a 3D scene). Solution: Use Flutter’s Texture widget or PlatformView to embed the 3D content. The Texture widget is ideal for this use case – the native side of the plugin renders into a GPU texture, which Flutter then composites into its widget tree. This allows layering: Flutter can draw widgets over or under the 3D texture as needed. The
flutter_gl
plugin uses this mechanism, where after drawing to an off-screen FBO, it callsupdateTexture()
to update the Flutter widget. One challenge is synchronization: ensuring the Flutter UI and the 3D render loop stay in sync with frames. Usually, locking the 3D refresh to Flutter’s frame clock (16.6ms for 60Hz) is sufficient. For user interactions, coordinate conversions are needed – e.g., mapping a touch on the Flutter widget to a ray in the 3D world for picking objects. This requires feeding Flutter gesture data into the 3D engine’s input system, which can be done through method channels or direct Dart calls into the engine API.
- Memory and Resource Management: Managing GPU resources across the FFI boundary can be complex. If the Dart side holds a reference to a texture or buffer allocated in native code, you need to ensure proper cleanup when a widget is disposed or an app is paused. Solution: Provide explicit methods to allocate and free resources in the API (for example, a method to destroy a 3D model or texture when no longer needed). Flutter’s lifecycle (e.g.,
dispose()
on widgets) can call into native to free resources. Using smart pointers or Rust’s ownership can help avoid leaks on the native side. Also consider the impact of hot-reload in Flutter (during development) – resources might need to be reinitialized after a reload.
- Package Size and Build Complexity: Including Vulkan and OpenGL support means shipping native libraries for multiple platforms, which can increase app bundle size. For instance, a Vulkan loader and validation layers on Android, or the size of the Filament library (~2-3 MB per architecture). Solution: Strive to keep the native library minimal – include only the necessary parts (strip debug symbols in release, avoid linking unused components). Use conditional imports or features to omit parts of the library on platforms where they aren’t needed (e.g., don’t include Vulkan-related code for iOS build if using a separate Metal path). Also, set up CI scripts to automate building the native components for all target platforms to reduce human error. Flutter’s build system can be configured to include prebuilt binaries or to run build scripts (with the native assets feature or Flutter plugin build hooks).
- Tooling and Debugging: Debugging across Dart and C++/Rust is more difficult than a pure Dart solution. One might encounter bugs that crash the app with no Dart stack trace (coming from the native side). Solution: Invest time in setting up good debugging tools. For C++ on Android/iOS, LLDB or GDB can be attached; for Rust, one can use
rr
or debug info through LLDB as well (Rust can be compiled with debug symbols). Logging is your friend: provide a way to get logs from the native engine (maybe piping them through a Dart callback or writing to a file). Additionally, use graphics debugging tools like RenderDoc for Vulkan/OpenGL or Xcode’s Metal debugger to inspect frames and catch API errors. During development, enabling graphics API validation layers (especially Vulkan’s validation) will catch mistakes in usage and provide meaningful feedback.
- Web-Specific Challenges: On Web, beyond just using WebGL, there are constraints like the single-threaded nature of JavaScript (though WebGL calls can happen on a Web Worker with OffscreenCanvas in modern browsers). Also, the browser security model might block certain capabilities. Solution: Use OffscreenCanvas and Web Workers for rendering if you need to parallelize away from the main UI thread on web. This is an advanced technique: you’d have a Web Worker running the WASM 3D engine and an OffscreenCanvas to do WebGL drawing, which can improve performance by not blocking the main thread (where Flutter’s DOM operations occur). If that’s too complex, ensure the WebGL content is efficient enough to run on the main thread without lag. Also consider feature detection in the browser: check for WebGL2 support, and provide a graceful message or lower-quality rendering if the user’s browser is outdated. Testing on multiple browsers (Chrome, Firefox, Safari) is needed, as each may have quirks in their WebGL implementations.
Implementing a cross-platform engine is a non-trivial undertaking, but each of these challenges has known solutions. The key is to design with flexibility in mind: abstract away platform differences, keep the Dart <-> native interface lean, and test often. By learning from existing projects (many of which encountered these issues), one can avoid common pitfalls.
Examples of Similar Projects and Architectures
Looking at how other projects structure their Flutter 3D solutions provides insight into best practices:
- Three_dart + flutter_gl Architecture: In this design, the 3D engine is written mostly in Dart (mirroring Three.js), and
flutter_gl
acts as the bridge to graphics APIs. Dart code creates a scene graph, materials, lights, etc., and when it issues a rendering command, internally it callsflutter_gl
(via FFI) which executes the corresponding OpenGL/WebGL call. Theflutter_gl
plugin manages an offscreen OpenGL context on mobile/desktop and a WebGL context on web, rendering into a texture. That texture is then displayed in a Flutter widget. This architecture is attractive for allowing Dart-level control and reuse of Three.js logic; however, performance depends on how efficientlyflutter_gl
can batch calls. The plugin mitigates overhead by providing a WebGL-like API so that Dart can make relatively high-level draw calls (e.g.,gl.drawElements(...)
) that the native side executes. Still, complex scenes might push Dart to its limits, making this approach suitable for moderately complex 3D, but perhaps not high-end 3D games.
- Flutter Scene (Impeller-based) Architecture: Flutter Scene takes a different route by leveraging Flutter’s own rendering backend (Impeller). Impeller uses Vulkan on Android, Metal on iOS, OpenGL as fallback, etc., all behind the scenes. Flutter Scene (which uses the experimental “Flutter GPU” API) taps into this system, meaning the Flutter engine is doing the heavy lifting. The Flutter GPU API likely provides Dart bindings to create buffers, issue draw calls, and compile shaders in a way that the Impeller backend can consume. In essence, Flutter’s engine becomes the renderer, and
flutter_scene
is a Dart-side wrapper to drive it. The benefit is performance and integration – it’s as if 3D is a first-class citizen in Flutter. Also, because Impeller is built with modern APIs, it automatically gains Vulkan and Metal support without the developer writing separate backends. The drawback is that this API is not officially stable; it requires Flutter master channel and works only on platforms Impeller supports (Android/iOS/macOS as of Flutter 3.7+, with Windows/Linux still using older renderers unless enabled). As Impeller becomes default everywhere, this approach could become the standard way to do Flutter 3D. Right now, it’s cutting-edge and might involve dealing with undocumented or changing APIs.
- Thermion (Flutter + Filament) Architecture: In Thermion’s approach, the heavy-duty rendering is done by the Filament engine (in C++), and Flutter is a host that displays Filament’s output and handles UI. The architecture is typically split into two parts: a Dart side (which provides a
ThermionViewer
API to the app) and a platform side (native code binding to Filament). When the Flutter app loads, the Thermion plugin initializes Filament (loading native libraries for the platform) and creates a rendering surface tied to a Flutter Texture. The app can then call methods likeloadGlb
(to load a 3D model) or move the camera via the Dart API, which under the hood call into the native code (via FFI) to manipulate the Filament scene. Each frame, Filament renders into the texture. This design cleanly separates concerns: Filament handles all rendering (using whatever API is optimal per platform: OpenGL on older Android, Metal on iOS, Vulkan on newer Android, etc.), while Dart is mainly an orchestrator. The inclusion of a WASM build for web means the same Filament C++ code is compiled to WebAssembly, and the Dart code can interact with it through JavaScript. That way, the high-level API remains consistent. This architecture offers high fidelity (Filament is a robust engine with PBR, global illumination, etc.) and leverages a proven engine. The complexity lies in the integration layer – ensuring the asynchronous nature of loading models and the multi-threading in Filament play nicely with Flutter’s single-threaded UI. Thermion’s success in displaying glTF models with PBR on Flutter across platforms is a strong proof of concept for this approach.
- Unity/Unreal Platform View Integration: A different architectural approach is treating the 3D engine as a “black box” that runs alongside Flutter. Unity, for example, can render to a texture or a native view that Flutter’s widget tree embeds. Communication between Flutter and the engine happens via message passing (method channels or Unity’s messaging). This is less of a library and more of an integration strategy. It’s suitable when one needs full engine capabilities (physics, terrain, etc.) and is willing to accept the heavyweight nature. The architecture is essentially two apps in one: Flutter handles UI and maybe app logic, while Unity/Unreal handles all graphics in its own world. The downside is limited interaction: e.g., you can’t easily overlay Flutter widgets on top of a Unity view with transparency – typically the Unity view will either be on top of Flutter or vice versa. Also, input events might need to be forwarded manually. This approach is proven (several apps use it), but it’s a different paradigm than a Flutter-centric library.
Comparison: The three_dart approach keeps everything in Dart (easy to use, but performance-limited), the Impeller approach keeps everything within Flutter’s engine (promising performance, but currently experimental), and the Filament approach offloads to an external engine (powerful, but complex integration). A hybrid library could even combine ideas: for example, use Dart for scene management and simple math, but perform all actual rendering in native code (a bit like how the Flutter Engine works for 2D). That would give a more Flutter-like API to the user (high-level, Dart-based), while preserving speed where it counts.
Feasibility, Tools, and Recommendations
Technical Feasibility: Building a cross-platform 3D library for Flutter is technically challenging but feasible – existing projects (though young) have shown it can be done. The availability of Flutter’s FFI, the performance of modern devices, and the adaptability of engines like Filament or frameworks like
flutter_gl
provide a foundation. The developer will need expertise in graphics programming (especially Vulkan, which has a steep learning curve) and a solid understanding of Flutter’s rendering lifecycle. One encouraging sign is Flutter’s own trajectory: with Impeller and Flutter WebAssembly support improving, the framework is becoming more amenable to custom rendering. By leveraging Vulkan for its performance and using OpenGL as a safety net, one can achieve wide platform coverage with high efficiency.Available Tools & Libraries: Leverage what’s already out there to avoid reinventing the wheel:
- flutter_gl: Useful if you decide to implement the engine in Dart. It saves you from writing the low-level GL bindings for each platform, and provides a unified interface. It’s a good starting point for quick experiments in Dart, though for Vulkan you’d need a different solution (perhaps an equivalent Flutter Vulkan binding if it exists, or writing one via FFI).
- Impeller/Flutter GPU: Keep an eye on Flutter’s official support. If Flutter GPU API becomes stable, using it might drastically simplify development – you’d get a cross-platform GPU context managed by Flutter and could write Dart code to draw 3D. This might, however, limit you to what Flutter exposes (which currently is geared towards rendering shapes for UI, not full scene management).
- Game engines and renderers: Decide if integrating an existing engine is better than writing from scratch. For example, Filament (with Thermion) already handles multi-platform rendering with Vulkan/Metal/GL. Godot engine (open source) could theoretically be embedded in a similar way (there was community interest in a Godot Dart interface). bgfx could be integrated for a lighter weight renderer. Using these could save years of development, letting you focus on the Flutter-specific parts. The trade-off is adapting to their APIs and possibly carrying extra features you don’t need.
- Language interoperability tools: For C++, check out Flutter’s official docs on using C libraries with FFI. For Rust, tools like
flutter_rust_bridge
orcxx
can facilitate binding. Android NDK and iOS toolchains will be needed to compile the native code; setting up a unified build (possibly via CMake or cargo with cross-compilation) is important.
Recommendations for Implementation:
- Start with a Prototype: Determine the core approach by prototyping a simple spinning cube on each platform. For instance, create a minimal Flutter plugin that opens a Vulkan surface on Android (falling back to GL) and a WebGL canvas on web, and renders a triangle. This will flush out platform integration issues early. You could use a simple shader and hard-coded vertices just to validate the pipeline.
- Choose Engine Architecture: Decide between a Dart-centric engine or a Native-centric engine. A Dart-centric engine (like three_dart) makes the API easier for Flutter developers (pure Dart usage) but may struggle with performance for large scenes. A native-centric engine (like using Filament or writing in C++/Rust) offers better performance and access to advanced graphics features, at the cost of more complex API (some async call bridging, etc.). Given the requirement for performance and efficiency, a native backend is recommended – perhaps a hybrid where Dart is used for scene composition and the native side for rendering routines.
- Use Vulkan where possible, but implement fallback path: Structure the code to attempt Vulkan initialization on startup. If it fails (catch the error or check support), initialize an OpenGL ES context instead. This could be encapsulated in a single engine initialization function. For example, on Android, try
vkCreateInstance
; if unavailable, use an EGL context. On Windows, try loading Vulkan via the loader, else use WGL/OpenGL. This logic can be hidden behind an abstractGraphicsContext
class. Ensure that shader code and asset formats are prepared for both APIs (you might need both SPIR-V and GLSL versions of your shaders – tools can convert one to the other, or use GLSL for GL and HLSL->SPIRV for Vulkan via DXC or glslang).
- Integrate with Flutter via Texture: Plan the Flutter widget that will display the 3D content. The simplest is a
Texture()
widget which is given an ID linked to the native texture. The plugin on each platform creates a texture (using Flutter’s texture registry) and renders into it. This allows you to create multiple 3D viewports if needed, just by creating multiple textures. Provide a Flutter-side controller class (e.g.,ThreeFlutterView
or similar) that manages this texture ID and offers methods likeonPan()
to handle user input,render()
to trigger frame draws (or start an automatic rendering loop). This controller can also manage the lifecycle (pause rendering when not visible, etc.).
- Focus on Performance Early: Use profiling and optimization techniques from day one. For example, if using FFI, design the API such that you don’t need to call 1000 functions per frame. It might be worth implementing a small command buffer in Dart: collect draw commands in Dart (cheaply, just as data), then send them in one FFI call to the native side to execute. This way, even a Dart-driven engine could batch its work. If using a native engine, try to offload computations like skeletal animation or particle updates to the native side as well, to avoid huge data transfers each frame. Remember the tip from
flutter_gl
: prefer using native allocated arrays to avoid copying when sending large vertex data.
- Learn from Community Projects: Examine the source code of projects like
flutter_gl
,flutter_scene
, or Thermion (if open source) for hints on implementation. For instance,flutter_scene
being Impeller-based might show how they handle coordinate systems or camera (likely similar to three.js with a PerspectiveCamera, etc.).three_dart
can serve as a guide for API design, since Three.js is very popular – adopting similar class names (Scene, Camera, Mesh, Material) could make it easier for developers to pick up your library. The community packages and their documentation can also highlight limitations they hit, so you can address them (for example, three_dart’s lack of Linux support suggests some issue with GL on Linux – possibly the need to include X11 or GLX context creation in flutter_gl).
- Documentation and API Design: Lastly, design the API to be Flutter-friendly. This means using Dart conventions, providing Widgets if appropriate (maybe a
SceneView
widget that internally uses the Texture, so the user doesn’t deal with IDs), and making it feel like an extension of Flutter. If the target audience is Flutter developers (who may not be graphics experts), hiding the complexity of Vulkan and OpenGL behind a simple interface is crucial. Offer sensible defaults (e.g., a default lighting and camera setup so a newbie can just load a model and see it). Also, document how to deploy to each platform, especially any extra steps (for instance, bundling shader files or requiring certain permissions).
In conclusion, implementing a cross-platform 3D library for Flutter “similar to Three.js” is an ambitious project that blends two worlds: high-performance graphics and Flutter UI development. By studying existing efforts and using a mix of WebGL for web, Vulkan for modern native platforms, and OpenGL as a fallback, one can achieve broad reach
. The use of C++ or Rust is recommended to meet performance demands, interfacing with Flutter via FFI for a seamless experience. As Flutter continues to evolve (with Impeller, WASM, and maybe future WebGPU support), the gap between Flutter and 3D graphics is closing. With careful planning and optimization, a Flutter 3D library can deliver rich, real-time 3D content across mobile, web, and desktop, opening the door for Flutter apps to incorporate complex visualizations, games, and AR/VR style experiences in the near future.
Sources:
- Flutter three_dart library (Three.js in Dart) – Pub.dev documentation
- flutter_gl OpenGL bridge for Flutter – GitHub README
- Flutter Scene 3D library – GitHub README
- Flame engine discussion on 3D support – GitHub Discussions
- Flutter Impeller engine documentation – Flutter.dev docs
- Reddit discussion on Filament (Thermion) for Flutter – Reddit r/FlutterDev
- GitHub issue on using Filament with Flutter via FFI – Filament GitHub issue
- Reddit thread on Vulkan vs OpenGL portability – Reddit r/vulkan